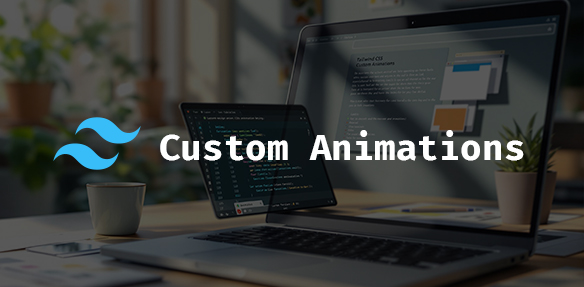
Want to create smooth animations effortlessly? Tailwind CSS makes it simple with pre-built utilities and customizable options. Here’s what you’ll learn:
- Core Tools: Use transition and animation classes like
animate-spin
ortransition-colors
for instant effects. - Custom Animations: Define unique keyframes in
tailwind.config.js
for tailored designs. - Performance Tips: Optimize animations with GPU-accelerated properties like
transform
andopacity
. - Advanced Techniques: Combine animations for layered effects and respect user preferences with
motion-safe
.
Tailwind lets you build animations quickly, optimize them for performance, and customize them to fit any project. Let’s dive in.
Tailwind Animation Basics
Let’s dive into the core mechanics of Tailwind’s animation tools and see how they can enhance your UI.
Basic Transition Commands
Tailwind provides simple utilities for adding transitions:
<button class="transition-colors duration-300 hover:bg-blue-600">
Hover color transition
</button>
Here’s how it works:
- The
transition
utility enables property changes. duration-*
sets how long the transition lasts.ease-*
adjusts the animation curve.
For better performance, stick to specific transitions like transition-colors
instead of transition-all
.
Property Type | Utility Class | Example Use Case |
---|---|---|
Color Changes | transition-colors | Button hover effects |
Size/Scale | transition-transform | Expanding cards |
Opacity | transition-opacity | Fade-in/out effects |
Position | transition-transform | Sliding menus |
Transitions vs Animations
Knowing when to use transitions versus animations can make your UI smoother and more efficient. Transitions are great for simple state changes, while animations handle more elaborate sequences.
<!-- Transition Example -->
<div class="transition-transform duration-200 hover:translate-x-4">
Smooth slide on hover
</div>
<!-- Animation Example -->
<div class="animate-spin h-8 w-8 border-t-2 border-blue-500 rounded-full">
Continuous rotation
</div>
Pre-built Animation Classes
Tailwind offers a range of pre-built animation classes that cover most common needs:
<div class="flex space-x-4">
<button class="animate-bounce bg-green-500 px-4 py-2">New!</button>
</div>
Here are two popular options:
animate-bounce
: Great for grabbing attention.animate-pulse
: Ideal for indicating loading states.
For more control, you can fine-tune animations using arbitrary values:
<div class="animate-bounce duration-[2000ms]">
Custom-timed bounce
</div>
These tools let you create sleek, responsive animations that elevate your design effortlessly.
Building Custom Animations
Pre-built classes are great for standard needs, but custom keyframes give you full control over animation details. Here’s how you can create animations tailored to your design.
Setting Up Custom Keyframes
To define custom animations, update your tailwind.config.js
file like this:
// tailwind.config.js
module.exports = {
theme: {
extend: {
// Define keyframes and their behavior
keyframes: {
glow: {
'0%, 100%': { boxShadow: '0 0 10px #ff00ff' },
'50%': { boxShadow: '0 0 20px #ff00ff' }
},
wave: {
'0%': { transform: 'rotate(0deg)' },
'10%': { transform: 'rotate(14deg)' },
'20%': { transform: 'rotate(-8deg)' },
'100%': { transform: 'rotate(0deg)' }
}
},
animation: {
glow: 'glow 1.5s infinite',
'waving-hand': 'wave 2s linear infinite'
}
}
}
}
These animations work perfectly with Tailwind’s utilities like duration-*
and delay-*
.
Using Custom Animation Classes
You can apply these animations directly in your HTML:
<div class="animate-glow duration-1000 delay-200">
Glowing element
</div>
<button class="animate-waving-hand hover:pause">
👋 Wave on hover
</button>
Want to combine animations? Use comma-separated keyframes:
// tailwind.config.js
theme: {
extend: {
animation: {
'card-entry': 'fadeIn 0.5s ease-out, slideUp 0.6s ease-in-out'
}
}
}
“When implementing custom animations, always consider the performance implications. Limit concurrent animations to less than 3 on mobile devices and use
transform
andopacity
properties whenever possible for smooth performance.” [2]
Making Animations Run Faster
You’ve crafted custom animations - now let’s make sure they perform well on all devices.
GPU vs CPU Properties
The secret to faster animations is choosing the right properties. GPU-accelerated properties like transform
and opacity
are much faster than CPU-dependent ones. Check out this comparison:
Property Type | Performance Impact |
---|---|
GPU-Accelerated | High |
CPU-Dependent | Low |
Tips for Better Mobile Performance
To optimize animations for mobile devices, pair Tailwind’s motion utilities with transform-based animations:
<div class="motion-reduce:animate-none md:animate-spin duration-150">
<!-- Animation optimized for performance -->
</div>
Tests show that transform-based animations are about 4x faster than those relying on layout changes [2].
Loading Animations Only When Needed
Efficient animation loading is just as important as creating them. Use the Intersection Observer API to lazy-load animations only when they enter the viewport:
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
entry.target.classList.add('animate-fadeIn');
}
});
});
// Apply to elements
document.querySelectorAll('.lazy-animate').forEach((el) => {
observer.observe(el);
});
This approach ensures your animations don’t unnecessarily impact performance when they’re not visible.
For testing, utilize Chrome DevTools’ Performance panel with CPU throttling enabled. It’s a great way to pinpoint any issues before going live [2].
Also, don’t overlook user preferences. Implement the prefers-reduced-motion
utility to respect users who prefer minimal motion:
<div class="animate-bounce motion-safe:animate-none">
<!-- Adjusts animation based on user settings -->
</div>
Advanced Animation Methods
Tailwind’s efficient foundation allows you to build complex, professional animations without sacrificing performance. Let’s explore some advanced techniques to take your motion design to the next level.
Multi-layer Animation Effects
Add depth and complexity by layering animations on nested elements:
<div class="relative group">
<div class="animate-bounce hover:scale-110">
<div class="animate-spin duration-300">
<img src="avatar.png" class="animate-pulse" />
</div>
</div>
</div>
For interactive elements, blend transform properties with custom timing for smooth transitions:
<button class="transition-all duration-300 ease-in-out transform hover:scale-110 hover:rotate-3 hover:bg-blue-600">
Interactive Button
</button>
Animation Testing Tools
Fine-tuning animations is easier with the right tools. Here’s a quick comparison of some must-have options:
Tool | Primary Use | Key Feature |
---|---|---|
Hoverify | Real-time inspection | Live animation adjustments |
Chrome DevTools | Performance analysis | Animation timeline debugging |
Motion One | Complex sequences | Visual animation editor |
Storybook | Component isolation | Focused component testing |
These tools work alongside Tailwind’s utilities, giving you detailed control over your animation workflow.
Custom Timing and Easing
Tailwind makes it simple to integrate custom easing functions. Add them to your tailwind.config.js
file for precise timing control:
module.exports = {
theme: {
extend: {
transitionTimingFunction: {
'in-expo': 'cubic-bezier(0.95, 0.05, 0.795, 0.035)',
'out-expo': 'cubic-bezier(0.19, 1, 0.22, 1)',
},
},
},
}
Need even more control? Define custom keyframes with exact timing and transitions:
keyframes: {
customFade: {
'0%': { opacity: 0, transform: 'translateY(10px)' },
'100%': { opacity: 1, transform: 'translateY(0)' },
},
},
animation: {
customFade: 'customFade 0.5s cubic-bezier(0.25, 0.1, 0.25, 1) forwards',
},
These techniques open up endless possibilities for creating polished, dynamic animations.
Summary
Tailwind CSS gives developers tools to build smooth, efficient animations while balancing ease of use and customization. Its animation approach stands out for three key reasons: optimized performance, flexible keyframe configurations, and quick implementation with utility classes.
Optimized Performance Tailwind ensures animations run smoothly and load quickly, delivering visually appealing results across all devices.
Flexible Customization Developers can fine-tune animations and transitions using the tailwind.config.js
file [1]. This method ensures consistent designs while allowing room for tailored motion effects to fit specific project needs.
Efficient Development Utility classes make it easy to implement animations quickly without compromising performance. This approach simplifies creating and managing even complex animations across different projects.