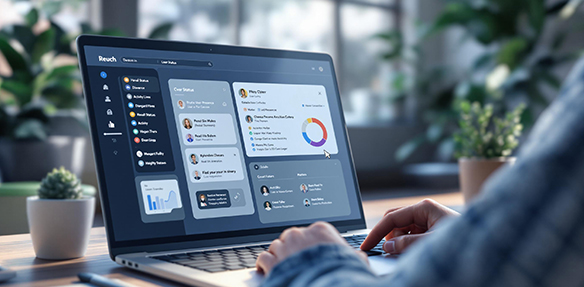
User presence features like real-time status updates, live cursors, and activity indicators make web apps more interactive and collaborative. They’re essential for apps like Google Docs, Discord, and telemedicine platforms. This guide explains how to implement these features using tools like WebSockets, Socket.io, or PubNub, while also addressing scaling challenges.
Key Takeaways:
-
Features: Live cursors (track cursor movements), activity indicators (show typing, speaking), and status updates (online/offline).
-
Technologies: Use WebSockets for custom setups, Socket.io for simplicity, or PubNub for enterprise solutions.
-
Scaling Tips: Use Redis for managing user data and heartbeat signals to maintain accuracy.
-
Code Snippet: Track user activity with the
Visibility API
:window.addEventListener('visibilitychange', () => { updatePresenceStatus(document.visibilityState === 'visible'); });
By integrating these features, you can create dynamic, real-time environments that enhance user engagement and collaboration.
What Is User Presence in Web Apps?
User presence refers to tracking and displaying users’ real-time actions and statuses in shared online environments. It’s the system that lets you see if someone is online, what they’re doing, and even where their cursor is moving - think Google Docs or Discord.
Key Features of User Presence
Here are the main components that make user presence work:
Feature | Description |
---|---|
Live Cursors | Lets you see where other users’ cursors are in real time (e.g., Google Docs). |
Activity Indicators | Displays user actions, like typing or speaking, to show engagement (e.g., Zoom participant status). |
Status Updates | Shows whether someone is online, offline, or away (e.g., Discord). |
These features rely on “heartbeat signals”, small periodic messages exchanged between the client and server to keep the connection stable and ensure status updates are accurate [1].
Why Add User Presence Features?
Adding user presence features can transform how people interact in your app. It makes collaboration smoother, improves communication, and creates a better overall experience by providing instant feedback and visual cues. For example:
- Telemedicine apps use user presence for virtual waiting rooms, allowing real-time patient monitoring [3].
- Collaborative tools like shared workspaces show when teammates are actively editing, typing, or contributing.
Real-time updates make interactions feel immediate and connected. Platforms like PubNub highlight how modern presence systems use advanced publish-subscribe models to ensure instant status updates across all users [1].
These essential components - live cursors, activity indicators, and status updates - are the foundation of user presence. They’ll also be your starting point for integrating this feature into your web app.
How to Add User Presence to Your Web App
Selecting Tools and Technologies
To enable real-time communication in your app, tools like WebSockets and Socket.io are essential. These technologies are widely used for building user presence features. Here’s a quick comparison:
Technology | Best For | Key Features |
---|---|---|
WebSockets | Custom implementations | Direct bidirectional communication |
Socket.io | Quick setup | Built-in presence management |
PubNub/Ably | Enterprise scale | Pre-built presence infrastructure |
Once you’ve chosen the right tool for your needs, the next step is setting up how you’ll manage user connections and track their presence.
Tracking User Connections and Disconnections
To track when users are online or offline, you’ll need event listeners on both the client and server. On the frontend, the Visibility API can help detect when users switch tabs or minimize their browser windows:
window.addEventListener('visibilitychange', () => {
updatePresenceStatus(document.visibilityState === 'visible');
});
Additionally, heartbeat signals are crucial. These periodic checks ensure the connection is still active and accurately reflect user activity. With a solid connection tracking system in place, you can move on to adding interactive features like live cursors and activity indicators.
Adding Live Cursors and Activity Indicators
Live cursors make collaboration more engaging. Here’s how you can implement them:
- Track and broadcast cursor movements: Use event listeners to capture cursor coordinates and send updates through WebSocket connections.
- Render smooth animations: Display other users’ cursor positions with animations for a seamless experience.
For activity indicators, frameworks like Phoenix LiveView (built for real-time Elixir apps) can simplify state updates and make implementation easier [2][4].
To keep performance in check, follow these tips:
- Reduce the frequency of cursor updates to avoid overloading the server.
- Use efficient data structures, such as hash maps or arrays, to store presence information.
- For apps with a large user base, consider distributed systems to handle the load effectively [3].
Tips for Building User Presence Features
Handling Large Numbers of Users
Managing user presence features at scale demands a well-thought-out approach to data handling and system design. One effective method is using Redis sets to store active user IDs and their status. This ensures quick lookups and updates with minimal delay.
Here are a couple of strategies to handle a growing user base effectively:
Strategy | How It Works | Why It Helps |
---|---|---|
Distributed Architecture | Use a publish-subscribe model with multiple servers | Balances server load and spreads connections |
Data Storage Optimization | Leverage Redis sets to track user presence | Supports 100K+ simultaneous users |
With these in place, you can focus on keeping the presence data accurate as your user base expands.
Using Heartbeat Signals for Accuracy
Accurate presence tracking becomes more challenging as user numbers grow. Heartbeat signals are a key tool for maintaining reliability. Here’s how to implement them effectively:
- Configure heartbeats to send updates every 15–30 seconds to strike a balance between accuracy and efficiency.
- Allow a buffer of 2–3 missed heartbeats before marking a user as offline.
- Monitor connection quality to manage temporary network issues.
“Real-time presence platforms leverage heartbeat signals to check the status of clients in real time, enhancing the accuracy of user presence data” [1].
This method ensures features like live cursors and activity indicators work smoothly, even during high traffic. It also enables your system to handle rapid status changes without compromising performance or accuracy [1].
Conclusion
Using real-time frameworks and APIs, developers can build dynamic platforms that encourage user interaction and create active communities. These tools help craft collaborative spaces where users can connect and communicate effortlessly.
The success of user presence features hinges on three main factors: choosing the right technologies, managing data efficiently, and ensuring accurate status tracking. Techniques like publish-subscribe models and heartbeat signals make presence detection scalable and precise. This makes them incredibly useful for a range of applications, such as telemedicine waiting rooms or online gaming platforms [3].
To maintain smooth performance as your application scales, it’s essential to consider how data storage and system architecture affect efficiency. Distributed systems and tools like Redis sets are excellent for managing presence data, offering a strong base for growing user numbers [1].
User presence features go beyond just showing whether someone is online. When done right, they transform static web apps into interactive, real-time environments where users can collaborate and engage. This not only boosts user engagement but also builds a stronger sense of community within your application [3].
As web applications continue to advance, integrating reliable and scalable presence features will become even more important. By applying the technical strategies and best practices covered here, you can create features that grow with your user base while maintaining top-notch performance and accuracy.