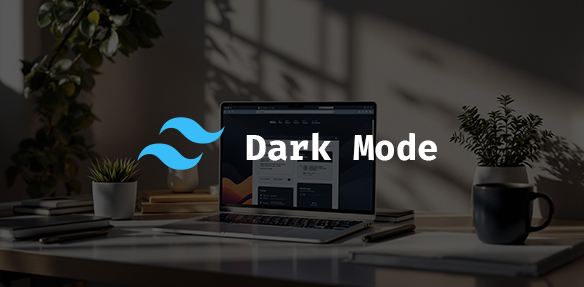
Dark mode is no longer optional - it’s a must-have for modern web design. Tailwind CSS makes implementing dark mode simple with two main approaches:
- Media Strategy: Automatically adapts to the user’s system preferences.
- Class Strategy: Allows users to toggle dark mode manually.
Here’s how to get started:
- Choose Your Approach: Decide between media (
darkMode: 'media'
) or class-based (darkMode: 'class'
) dark mode in yourtailwind.config.js
file. - Apply Styles: Use the
dark:
prefix to define styles for dark mode, e.g.,bg-white dark:bg-gray-800
. - Add a Toggle: For class-based dark mode, use JavaScript to let users switch themes and save their preferences in local storage.
Quick Comparison Table:
Feature | Media Strategy | Class Strategy |
---|---|---|
How It Works | Follows system preferences | User-controlled toggle |
Best For | Static websites | Interactive web applications |
Configuration | darkMode: 'media' | darkMode: 'class' |
Tailwind CSS’s flexibility ensures your dark mode setup is both user-friendly and accessible, with options to customize colors, add smooth transitions, and meet contrast standards.
Setting Up Dark Mode in Tailwind CSS
Here’s how you can configure dark mode in Tailwind CSS.
Choose Your Dark Mode Approach
Tailwind CSS offers two ways to implement dark mode, depending on your needs:
Method | Description | Best For | Configuration |
---|---|---|---|
Media Strategy | Syncs with the user’s system preferences | Static or content-focused websites | darkMode: 'media' |
Class Strategy | Lets you toggle dark mode manually using classes | Web applications with user preferences | darkMode: 'class' |
The media strategy works well for static sites that follow system settings, while the class strategy is ideal for apps where users can toggle dark mode themselves. Choose one and configure Tailwind accordingly.
Basic Dark Mode Setup
Follow these steps to enable dark mode:
1. Update Tailwind Configuration
Modify your tailwind.config.js
file like this:
module.exports = {
darkMode: 'class', // Use 'media' for system-based dark mode
theme: {
extend: {
colors: {
darkBackground: '#1a202c',
darkText: '#f7fafc',
},
},
},
}
2. Add Base Styling
Use the following HTML structure to apply light and dark mode styles:
<div class="bg-white dark:bg-darkBackground text-black dark:text-darkText">
<h1 class="text-2xl font-bold">Dynamic Content</h1>
<p class="mt-4">This content adapts to dark mode automatically</p>
</div>
For class-based dark mode, you can enable it with JavaScript:
// Check user preference and enable dark mode
if (localStorage.getItem('darkMode') === 'true') {
document.documentElement.classList.add('dark');
}
If you’re using Flowbite components, dark mode support is built-in through its plugin, making it easier to integrate pre-designed elements.
Accessibility and Customization
Ensure your light and dark mode designs comply with WCAG contrast ratios (4.5:1 for normal text, 3:1 for large text). For custom dark mode colors, you can extend your theme like this:
theme: {
extend: {
colors: {
dark: {
primary: '#1e293b',
secondary: '#334155',
accent: '#60a5fa',
},
},
},
}
With this setup, you can refine your styles and add a toggle to let users switch between light and dark modes dynamically.
Dark Mode Styling Guide
This guide dives into specific styling techniques to enhance your dark mode setup.
Tailwind CSS makes dark mode styling straightforward with its dark:
variant. Here’s how to use it effectively.
Applying Dark Mode Classes
You can add dark mode styles by prefixing any utility class with dark:
. For example:
<div class="bg-white dark:bg-gray-800 text-gray-900 dark:text-gray-100">
<h1 class="text-2xl font-bold text-blue-600 dark:text-blue-400">
Dynamic Heading
</h1>
<p class="mt-4 text-gray-700 dark:text-gray-300">
Content that works seamlessly in both light and dark modes
</p>
</div>
For interactive elements, ensure they remain clear and functional in dark mode:
Element Type | Light Mode | Dark Mode | Purpose |
---|---|---|---|
Primary Buttons | bg-blue-500 | dark:bg-blue-600 | Call-to-action buttons |
Secondary Elements | bg-gray-200 | dark:bg-gray-700 | Cards and panels |
Text Input | bg-white | dark:bg-gray-800 | Form fields |
Once the basics are in place, fine-tune your color palette for a polished dark mode look.
Customizing Dark Mode Colors
Take your design further by creating custom colors in your configuration. Make sure to maintain good contrast for readability.
<article class="bg-white dark:bg-darkBackground">
<h2 class="text-gray-900 dark:text-darkText">Main Heading</h2>
<p class="text-gray-700 dark:text-gray-300">Article content with proper contrast</p>
</article>
These adjustments will help ensure your dark mode styling feels cohesive and user-friendly.
Create a Dark Mode Switch
Adding a dark mode toggle lets users control their viewing experience, improving accessibility and usability.
JavaScript Toggle Implementation
Here’s how to create a functional toggle button for switching between light and dark modes:
<button id="theme-toggle" class="p-2 rounded-lg hover:bg-gray-100 dark:hover:bg-gray-700">
<svg id="theme-toggle-dark-icon" class="w-5 h-5 hidden">
<!-- Moon icon SVG -->
</svg>
<svg id="theme-toggle-light-icon" class="w-5 h-5 hidden">
<!-- Sun icon SVG -->
</svg>
</button>
Now, add JavaScript to handle the theme switching logic:
const themeToggleBtn = document.getElementById('theme-toggle');
const themeToggleDarkIcon = document.getElementById('theme-toggle-dark-icon');
const themeToggleLightIcon = document.getElementById('theme-toggle-light-icon');
themeToggleBtn.addEventListener('click', function() {
// Toggle icons
themeToggleDarkIcon.classList.toggle('hidden');
themeToggleLightIcon.classList.toggle('hidden');
// Toggle dark mode
document.documentElement.classList.toggle('dark');
// Save preference in local storage
const isDark = document.documentElement.classList.contains('dark');
localStorage.setItem('color-theme', isDark ? 'dark' : 'light');
});
This code ensures users can switch themes seamlessly.
Store User Dark Mode Settings
To make the theme selection persist across sessions, extend the functionality by checking and applying the saved preference:
// Check saved preference or system setting
if (localStorage.getItem('color-theme') === 'dark' ||
(!('color-theme' in localStorage) &&
window.matchMedia('(prefers-color-scheme: dark)').matches)) {
document.documentElement.classList.add('dark');
themeToggleLightIcon.classList.remove('hidden');
} else {
themeToggleDarkIcon.classList.remove('hidden');
}
Enhancing Accessibility
When designing the toggle button, include these features for better usability:
Feature | Implementation | Purpose |
---|---|---|
Keyboard Navigation | tabindex="0" | Allows keyboard users to interact |
ARIA Labels | aria-label="Toggle dark mode" | Adds context for screen readers |
Visual Feedback | hover:bg-gray-100 | Signals interactivity to users |
Place the dark mode toggle in a consistent and visible spot, like the navigation bar or footer, so users can easily find it.
Dark Mode Component Design
Designing components for dark mode requires attention to detail and a focus on usability. Here’s how you can achieve this using Tailwind CSS.
Component-Specific Dark Styles
Take this card example, which applies dark mode styling directly to the component. It balances clarity and usability:
<div class="bg-white dark:bg-gray-800 rounded-lg p-6 shadow-lg">
<h3 class="text-gray-900 dark:text-white text-xl font-semibold">
Card Title
</h3>
<p class="text-gray-600 dark:text-gray-300 mt-2">
Card content
</p>
<button class="mt-4 bg-blue-500 dark:bg-blue-600 text-white px-4 py-2 rounded
hover:bg-blue-600 dark:hover:bg-blue-700
focus:ring-2 focus:ring-blue-500 dark:focus:ring-blue-400">
Action Button
</button>
</div>
Here’s a quick reference for color choices to maintain consistency in light and dark modes:
Element Type | Light Mode | Dark Mode | Purpose |
---|---|---|---|
Primary Buttons | bg-blue-500 | dark:bg-blue-600 | Highlight key actions |
Text Input | bg-white | dark:bg-gray-700 | Improve input visibility |
Borders | border-gray-200 | dark:border-gray-600 | Separate content visually |
Icons | text-gray-600 | dark:text-gray-300 | Maintain visual hierarchy |
These styles ensure your components look great and function well in both light and dark modes.
Dark Mode Accessibility Standards
Accessibility is just as important as aesthetics. Here’s an example of how to ensure your components meet accessibility requirements:
<div class="prose dark:prose-dark">
<p class="text-base text-gray-800 dark:text-gray-200 leading-relaxed">
Maintain a contrast ratio of at least 4.5:1 for text.
</p>
<a class="text-blue-600 dark:text-blue-400 hover:underline
focus:outline-none focus:ring-2 focus:ring-blue-500">
Provide clear focus states for links.
</a>
</div>
When working on more advanced interfaces like tables or charts, ensure they remain accessible:
<table class="w-full border-collapse bg-white dark:bg-gray-800">
<thead class="bg-gray-50 dark:bg-gray-700">
<tr>
<th class="p-4 text-left text-gray-900 dark:text-gray-100">Header</th>
</tr>
</thead>
<tbody class="divide-y divide-gray-200 dark:divide-gray-600">
<tr class="hover:bg-gray-50 dark:hover:bg-gray-700">
<td class="p-4 text-gray-700 dark:text-gray-300">Content</td>
</tr>
</tbody>
</table>
Summary
Tailwind CSS makes it easy to create visually appealing and accessible web apps with dark mode. Its straightforward dark
variant and customizable settings allow developers to choose between system-based (media
) or manual (class
) setups. This works perfectly with Tailwind’s detailed component styling.
With Tailwind, you can control dark mode styling at the component level. By using the dark:
prefix, developers can style specific elements while ensuring designs remain consistent and easy to read in low-light settings.
Here’s a quick breakdown of Tailwind’s dark mode features:
Feature | How It Works | Why It Helps |
---|---|---|
Configuration | darkMode in tailwind.config.js | Centralized control for dark mode |
Styling Control | dark: prefix classes | Fine-tuned customization |
Color Management | Extended color palette | Maintains brand consistency |
Tailwind’s dark mode goes beyond simply flipping colors. By configuring tailwind.config.js
, teams can design dark themes that match brand styles and meet accessibility needs. This setup also makes it easier to update dark mode styles across an entire project.
FAQs
How to implement dark mode using Tailwind?
Here’s a quick overview of how to set up dark mode with Tailwind CSS, based on the steps outlined earlier.
Tailwind CSS supports two approaches for dark mode, and the class-based method is often preferred for its flexibility. Start by configuring your tailwind.config.js
file like this:
module.exports = {
darkMode: 'class',
theme: {
// your theme configuration
}
}
Once set up, you can use the dark:
prefix to define styles for dark mode. Here’s a simple example:
Element Type | Light Mode Class | Dark Mode Class | Effect |
---|---|---|---|
Background | bg-white | dark:bg-gray-800 | Background changes to dark gray |
Text | text-gray-900 | dark:text-white | Text switches to white |
Border | border-gray-200 | dark:border-gray-600 | Borders turn darker |
For toggling between themes, use a script like this:
const darkModeToggle = () => {
if (document.documentElement.classList.contains('dark')) {
document.documentElement.classList.remove('dark');
localStorage.setItem('color-theme', 'light');
} else {
document.documentElement.classList.add('dark');
localStorage.setItem('color-theme', 'dark');
}
}
Tips for a better experience:
- Ensure contrast ratios meet WCAG accessibility standards.
- Add CSS transitions for smoother theme changes.
- Save the user’s theme preference in local storage.
- Test dark mode across all major browsers to avoid compatibility issues.